OK so I've decided to try to do this myself so I can see the process, the only difference is I used Portainer to do a lot of the deployments instead of using the native which lets me easily use docker compose but you should be able to do this as well if you have access to the shell (since this would make things SOOO much easier).
So if you do have shell info, make a folder somewhere and create a docker compose file with this content:
Code:
# https://docs.docker.com/compose/environment-variables/
version: "2.4"
services:
postgres:
image: postgres:${POSTGRES_IMAGE_TAG}
restart: ${RESTART_POLICY}
security_opt:
- no-new-privileges:true
pids_limit: 100
read_only: true
tmpfs:
- /tmp
- /var/run/postgresql
volumes:
- ${POSTGRES_DATA_PATH}:/var/lib/postgresql/data
environment:
# timezone inside container
- TZ
# necessary Postgres options/variables
- POSTGRES_USER
- POSTGRES_PASSWORD
- POSTGRES_DB
mattermost:
depends_on:
- postgres
image: mattermost/${MATTERMOST_IMAGE}:${MATTERMOST_IMAGE_TAG}
restart: ${RESTART_POLICY}
security_opt:
- no-new-privileges:true
pids_limit: 200
read_only: ${MATTERMOST_CONTAINER_READONLY}
tmpfs:
- /tmp
ports:
- ${APP_PORT}:8065
- ${CALLS_PORT}:${CALLS_PORT}/udp
- ${CALLS_PORT}:${CALLS_PORT}/tcp
volumes:
- ${MATTERMOST_CONFIG_PATH}:/mattermost/config:rw
- ${MATTERMOST_DATA_PATH}:/mattermost/data:rw
- ${MATTERMOST_LOGS_PATH}:/mattermost/logs:rw
- ${MATTERMOST_PLUGINS_PATH}:/mattermost/plugins:rw
- ${MATTERMOST_CLIENT_PLUGINS_PATH}:/mattermost/client/plugins:rw
- ${MATTERMOST_BLEVE_INDEXES_PATH}:/mattermost/bleve-indexes:rw
# When you want to use SSO with GitLab, you have to add the cert pki chain of GitLab inside Alpine
# to avoid Token request failed: certificate signed by unknown authority
# (link: https://github.com/mattermost/mattermost-server/issues/13059 and https://github.com/mattermost/docker/issues/34)
# - ${GITLAB_PKI_CHAIN_PATH}:/etc/ssl/certs/pki_chain.pem:ro
environment:
# timezone inside container
- TZ
# necessary Mattermost options/variables (see env.example)
- MM_SQLSETTINGS_DRIVERNAME
- MM_SQLSETTINGS_DATASOURCE
# necessary for bleve
- MM_BLEVESETTINGS_INDEXDIR
# additional settings
- MM_SERVICESETTINGS_SITEURL
# If you use rolling image tags and feel lucky watchtower can automatically pull new images and
# instantiate containers from it. https://containrrr.dev/watchtower/
# Please keep in mind watchtower will have access on the docker socket. This can be a security risk.
#
# watchtower:
# container_name: watchtower
# image: containrrr/watchtower:latest
# restart: unless-stopped
# volumes:
# - /var/run/docker.sock:/var/run/docker.sock
Make an .env file within the same directory with the following content:
Code:
DOMAIN=domain.youare.using
TZ=UTC
RESTART_POLICY=unless-stopped
POSTGRES_IMAGE_TAG=13-alpine
POSTGRES_DATA_PATH=/data/mattermostpostgresql/data
POSTGRES_USER=mmuser
POSTGRES_PASSWORD=mmuser_password
POSTGRES_DB=mattermost
CALLS_PORT=8449
MATTERMOST_CONFIG_PATH=./volumes/app/mattermost/config
MATTERMOST_DATA_PATH=./volumes/app/mattermost/data
MATTERMOST_LOGS_PATH=./volumes/app/mattermost/logs
MATTERMOST_PLUGINS_PATH=./volumes/app/mattermost/plugins
MATTERMOST_CLIENT_PLUGINS_PATH=./volumes/app/mattermost/client/plugins
MATTERMOST_BLEVE_INDEXES_PATH=./volumes/app/mattermost/bleve-indexes
MM_BLEVESETTINGS_INDEXDIR=/mattermost/bleve-indexes
MATTERMOST_IMAGE=mattermost-team-edition
MATTERMOST_IMAGE_TAG=9.1
MATTERMOST_CONTAINER_READONLY=false
APP_PORT=8065
MM_SQLSETTINGS_DRIVERNAME=postgres
MM_SQLSETTINGS_DATASOURCE=postgres://${POSTGRES_USER}:${POSTGRES_PASSWORD}@postgres:5432/${POSTGRES_DB}?sslmode=disable&connect_timeout=10
MM_SERVICESETTINGS_SITEURL=https://${DOMAIN}
You can change the path to wherever like I had (see below for example)
Code:
MATTERMOST_CONFIG_PATH=/data/mattermost/config
MATTERMOST_DATA_PATH=/data/mattermost/data
MATTERMOST_LOGS_PATH=/data/mattermost/logs
MATTERMOST_PLUGINS_PATH=/data/mattermost/plugins
MATTERMOST_CLIENT_PLUGINS_PATH=/data/mattermost/client/plugins
MATTERMOST_BLEVE_INDEXES_PATH=/data/mattermost/bleve-indexes
Start the docker compose by running
After that you'll want to update the permission by running the following:
Code:
sudo chown -R 2000:2000 ./volumes/app/mattermost
At this point you can restart the mattermost container or bring down the stack then start it back up.
Congrats you are half way there!
Now within Plesk, make a new sub domain of the same name you've set for DOMAIN. Go into the domain go to Hosting & DNS, then Apache & nginx.
Now disable Proxy mode under Nginx Settings so Apache isn't being used, then for additional nginx directive paste in the following:
NGINX:
location ~ /api/v[0-9]+/(users/)?websocket$ {
proxy_set_header Upgrade $http_upgrade;
proxy_set_header Connection "upgrade";
proxy_set_header Host $http_host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
proxy_set_header X-Frame-Options SAMEORIGIN;
proxy_buffers 256 16k;
proxy_buffer_size 16k;
client_body_timeout 60;
send_timeout 300;
lingering_timeout 5;
proxy_connect_timeout 90;
proxy_send_timeout 300;
proxy_read_timeout 90s;
proxy_pass http://localhost:8065;
}
location / {
proxy_set_header Connection "";
proxy_set_header Host $http_host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
proxy_set_header X-Frame-Options SAMEORIGIN;
proxy_buffers 256 16k;
proxy_buffer_size 16k;
proxy_read_timeout 600s;
proxy_http_version 1.1;
proxy_pass http://localhost:8065;
}
From there, you're basically done (don't forget to go to the dashboard of your domain and issue yourself a SSL from Let's Encrypt). Now go to the site and register yourself an account and you're done!
Please note that the environment variables I showed above is a mixture of the default settings and settings I've set (for example, CALLS_PORT I've changed) so I would recommend that you change the username and password for the
POSTGRES_USER and
POSTGRES_PASSWORD.
If you want to do everything through Plesk panel's native docker extension here's what it looks like (you can ignore the PATH (and basically anything that is not listed in the ENV above) variable since that will be auto generated anyways).
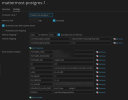